This article will go over the lifecycle hooks in React. Our clock was mounted using componentDidMount in the last article, which is a lifecycle loops. React provides three key phases for the entire life cycle of each component, which you can monitor and manage. The three phases are mounting, updating, and unmounting.
Mounting refers to adding components into the document object model or DOM. Hence ComponentWillMount() / componentDidMount() is the first life cycle hook. Our previous article made use of this lifecycle hook. We have two different hooks (or functions) that we can define to run functionality after it has been mounted. One is called immediately before the component is due for mounting on the page, and the other is called immediately following the component's mounting. This is useful for tasks such as retrieving data to populate a component. Let's take an example where we would like to show GitHub events using our activity tracker. These events should only be loaded when the data is ready to be rendered. In order to display the activities, we will edit the Content component to send a request to the GitHub events API and utilize the result. we'll want to make an HTTP request when the component is getting ready to be mounted (or just after it mounts). By defining the function componentWillMount() (or componentDidMount() ) in our component, React runs the method just before it mounts in the DOM. This is a perfect spot for us to add a GET request.
The next phase in the lifecycle is when a component is updated.A component is updated whenever there is a change in the component's state or props.The lifecycle hooks for this are componentWillUpdate() / componentDidUpdate(). Sometimes we'll want to update some data of our component before or after we change the actual rendering. For example, suppose we want to execute a function to initialize the rendering or a function when a component's props change. The componentWillUpdate() function is an acceptable hook for preparing our component for a change (as long as we do not call this.setState() to handle it (this will result in an infinite looping). But The componentWillReceiveProps() lifecycle hook will be a more common lifecycle hook used in this sceanrio.This is the first method that will be called when a component is going to receive a new set of props. To use this lifecycle hook in our timeline app, we may include a refresh button in our activity list, allowing users to request an update of the GitHub events API. Using this new prop (the requestRefresh prop), we can update the activities from our state object when it changes value.
The next phase of the lifecycle occurs when a component is removed from the DOM, also known as unmounting in React. Before the component is unmounted, React will call the componentWillUnmount() function. This is the time to handle any clean-up events that may be required, such as clearing timeouts and data. We need to clear the timeout we defined in the setTimer() function on the component when our clock is about to be unmounted. Adding the componentWillUnmount() function does this necessary cleanup.
As we have now understood a few lifecycle hooks, Let's Implement these in our timeline and clock code. First, we will work on the coding for the timeline. We will make our component stateful by assigning this.state to an object in the constructor. We'll set loading to false because we'll also be implementing a refresh button.
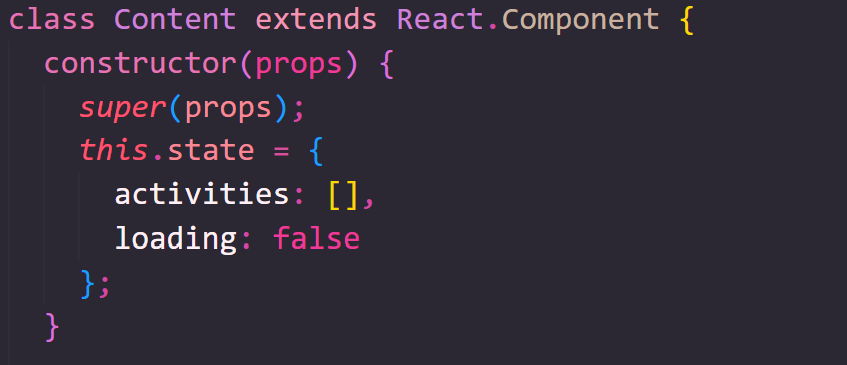
The next step is to make a small change to our ActivityItem component to match the new structure of our activity objects. Additionally, we transform the dates into a human-friendly string using the Moment.js library. We modified a few properties in ActivityItem and inserted this script tag.

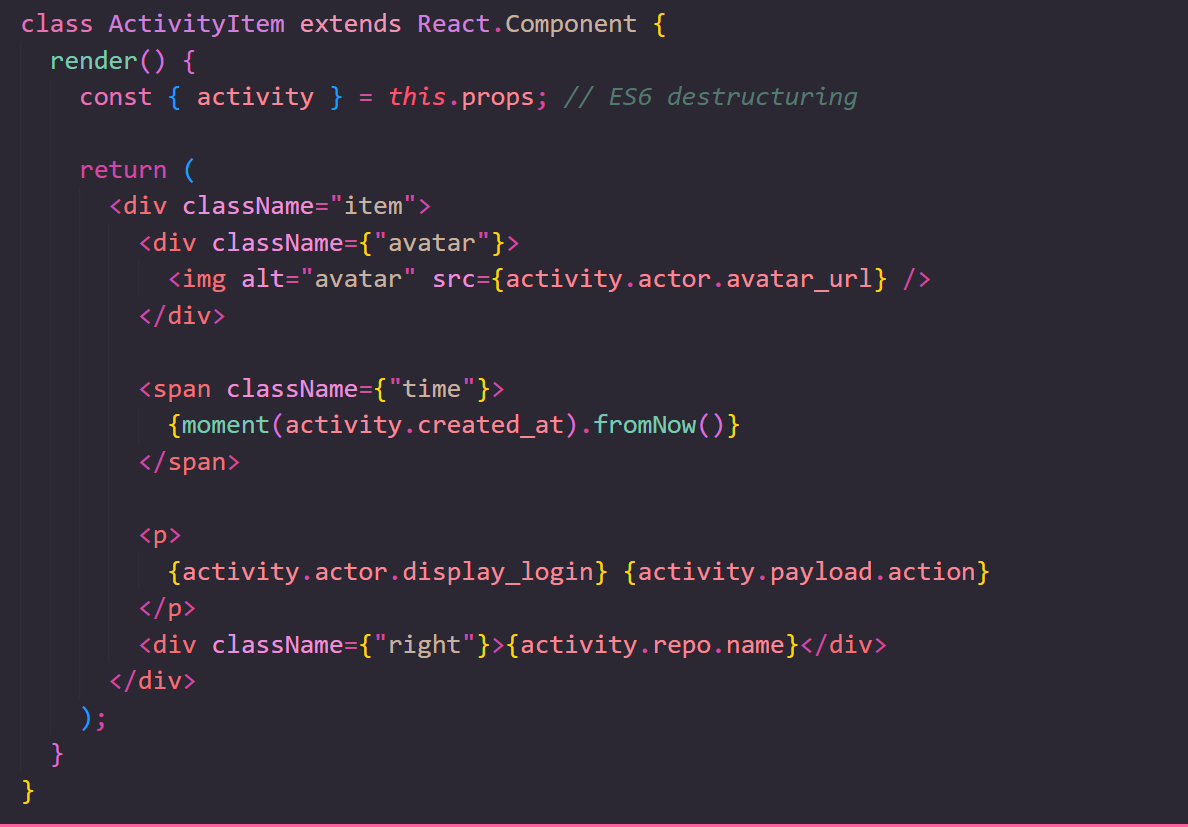
Let's include a refresh button now. We'll ask the component to reload its data using the componentWillReceiveProps() hook.To tell the Content component to refresh, the Refresh boolean prop is used. I have also added a footer Component.
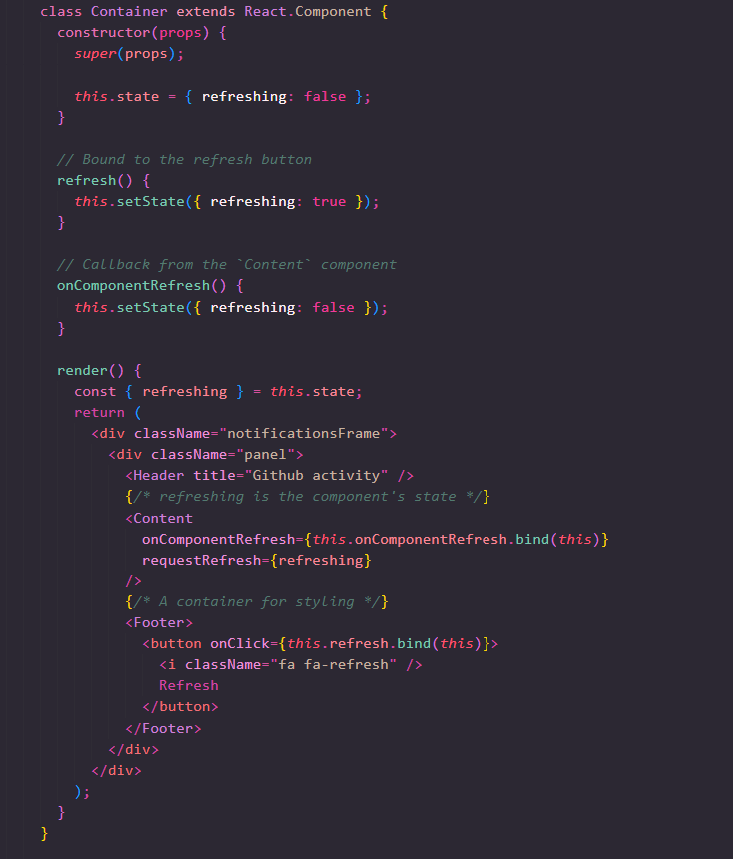

When the value of our state object changes, we can use this new prop—the requestRefresh prop—to update the activities. To update the activities, we will use the lifecycle hooks componentWillMount and componentwillreceiveProps.
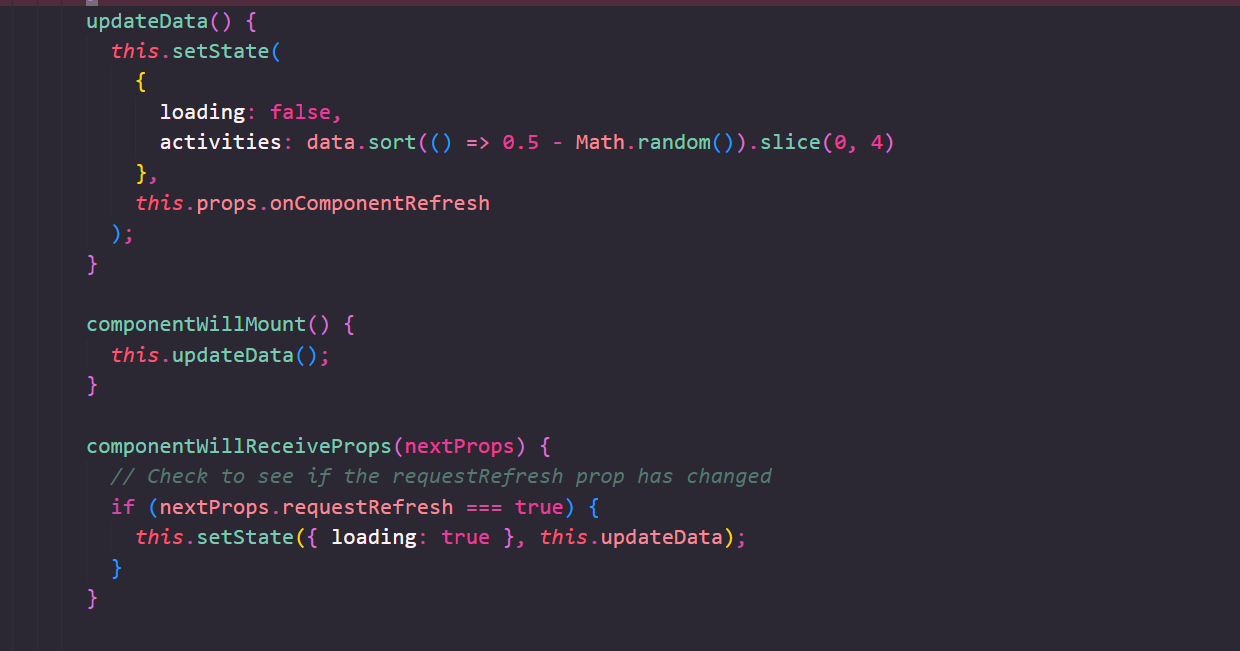
We are going to utilize the lifecycle hook componentWillUnmount() in our Timer. When we unmount our clock, we should clear the timeout that we defined in the component's setTimer() function. The componentWillUnmount() function handles the necessary cleanup. The unmount hook will be as follows.
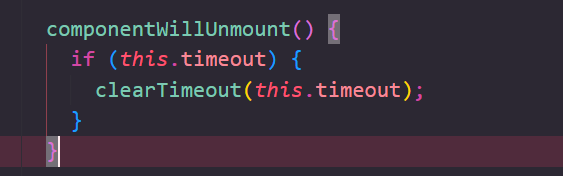
These are some of the lifecycle hooks present in the React framework. We'll be using these frequently as we develop our react apps.In the next article, we will create proptypes, style our components, and add interaction to them. The entire code for the article is available on my GitHub.
« Read Previous Article in this Series Read Next Article in this Series »